Deploy Docker image on Heroku
Docker takes away repetitive tasks and is used throughout the development lifecycle for fast development
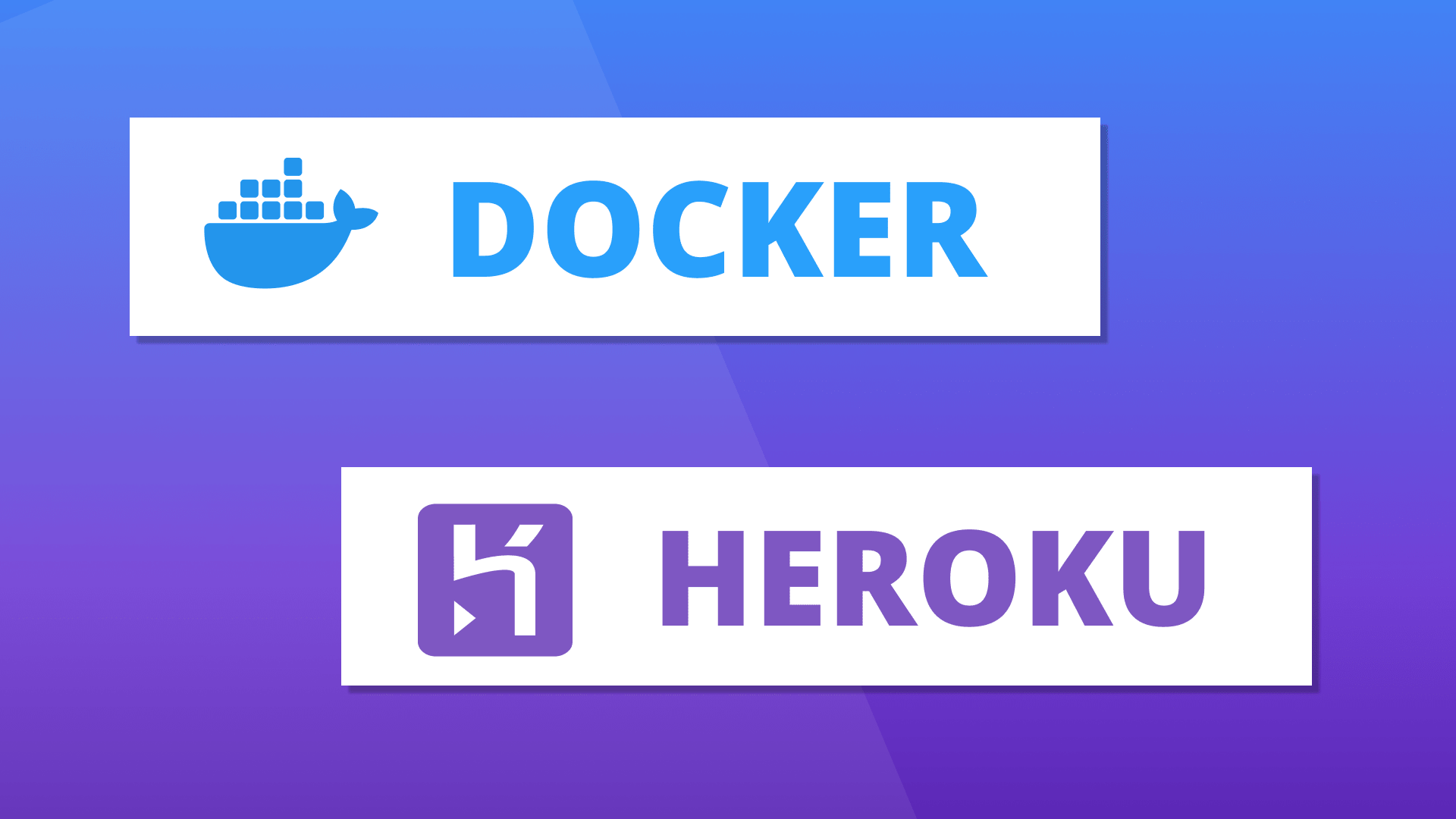
Dockerfile
The most important file for docker image that defines instructions how to deploy an application. For this tutorial, I will be using a Angular project but this method can be applied to pretty much any node.js application. First create a Dockerfile
in root directory of the project.
$ touch Dockerfile
Dockerfile instructions
For this project, I will be using node:14.1-alpine
image as base image. Feel free to explore other docker images as well. Moving on, You can set node env as production with ENV
command and move into directory by WORKDIR
Dockerfile
FROM node:14.1-alpine
ENV NODE_ENV=production
WORKDIR /app
Now, COPY
the package.json
and package-lock.json
from root directory of project to root directory of /app
. (because we WORKDIR
into /app
on 2nd step so we will use current directory symbol (.) ). Now installing node dependencies with production flag to ignore installing devDependencies
. After COPYing all files from our angular project into docker image we can write CMD
command which will execute the starting script of your application.
Dockerfile
FROM node:14.1-alpine
ENV NODE_ENV=production
WORKDIR /app
COPY package*.json ./
RUN npm install --production
COPY . .
CMD ["npm", "start"]
Dockerignore
Notice one step before CMD
we copied all files into Docker image which includes node_modules
folder as well. We can ignore node_modules
just like .gitignore
by creating .dockerignore
file in the root directory of project.
.dockerignore
node_modules
Heroku setup
If your machine does not have Heroku CLI installed, you can download it from their official website. Create a new Heroku app through its cli and set stack to container.
$ heroku login
$ heroku create <your-app-name>
$ heroku stack:set container -a <your-app-name>
Uploading image to Heroku
At this point, we can push our project into heroku container and release it to finish deployment process.
$ heroku container:push web -a <your-app-name>
$ heroku container:release web -a <your-app-name>
Heroku logs
You can see rest of the logs by heroku logs command for your app. And that is it, your application is dockerized and should be accessible on your-app-name.herokuapp.com
$ heroku logs -t -a <your-app-name>